#tag
基本知识的笔记
method方法绑定的this
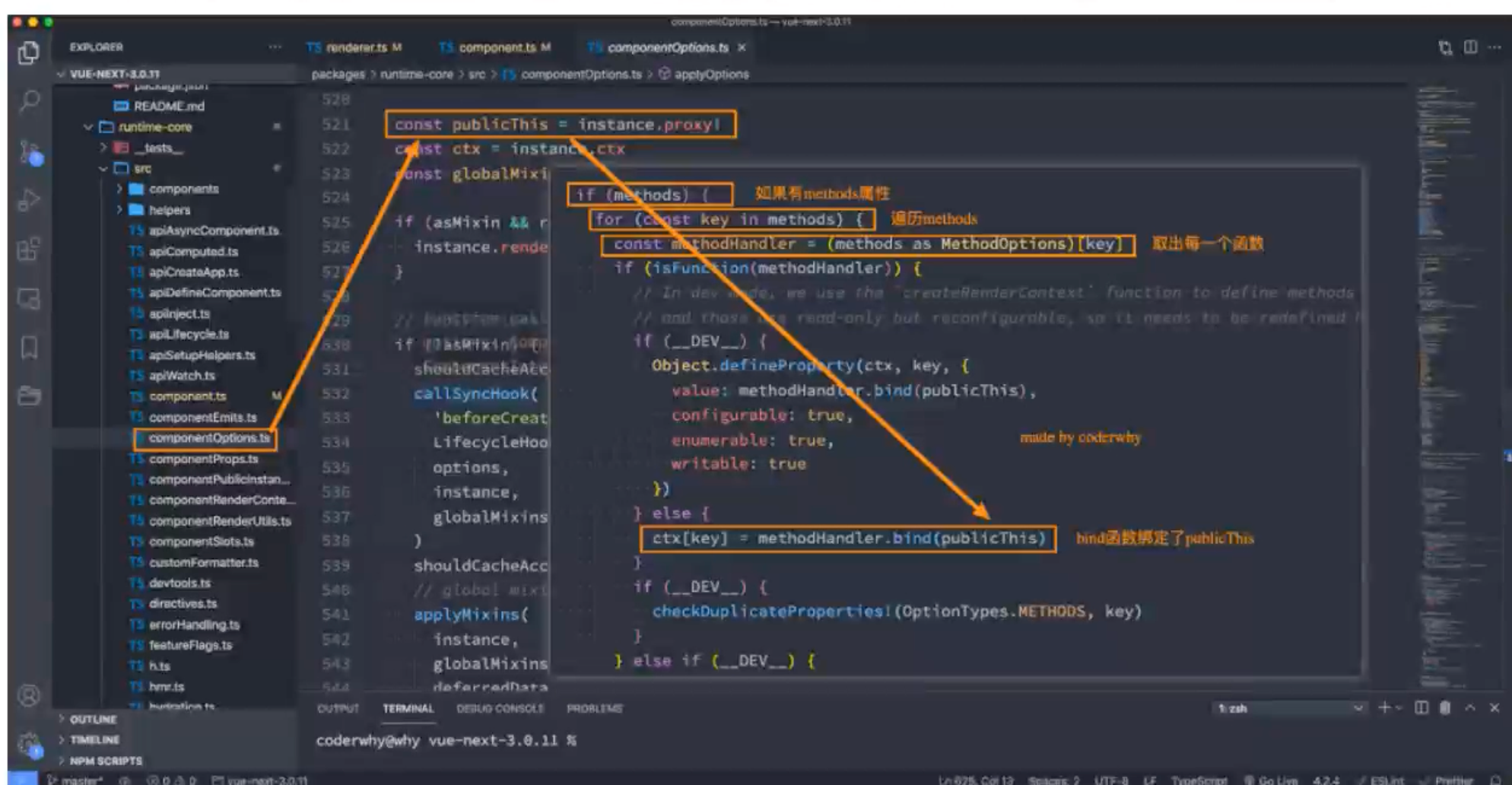
mustache语法
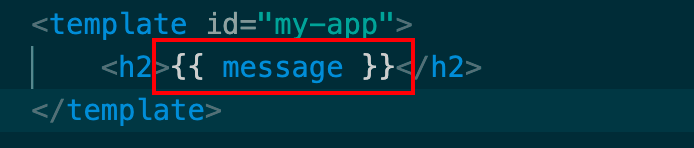
语法使用
常用指令
v-once
只会渲染一次
| <template id="my-app">
<h2>{{ message }}</h2>
<h2 v-once>{{ message }}</h2>
<button @click="changeMsg">改变</button>
</template>
|
1 2 3 4 5 6 7 8
| methods: {
changeMsg() {
this.message = ((Math.random() * 100000) + '').split(10).join('')
} }
|
每次点击改变
按钮时,v-once指令的当前元素与子元素都不会重新渲染
v-text
1 2
| <h2>{{message}}</h2> <h2 v-text="message"></h2>
|
上下代码,html的效果一致
v-html
1 2 3 4 5 6 7
| <template id="my-app">
<h2>{{message}}</h2>
<div v-html="htmlParam"></div>
</template>
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| ```data() {
return {
message: "Hello World",
htmlParam: `
<h1>Hello Vue</h1>
` } }
|
使用v-html解析出html标签的显示,若使用v-text会导致html标签也跟着显示出来,没有进行解析
v-pre
v-pre会跳过元素和子元素的编译过程,显示原始的Mustache标签
1 2 3 4
| <template id="my-app"> <h2>{{message}}</h2> <div v-pre>{{data}}</div> </template>
|
vue optionAPI的data中也没有定义data
这个变量
console中没有报错,证明使用v-pre后vue没有去解析Mustache语法的内容
v-bind
一些注意点
1
| <div :style="{color: colorParam, 'font-size': fontParam}">test</div>
|
对于一些有-
的css属性,可以使用驼峰形式也可以使用-
,如:fontSize
与font-size
,但是使用-
的话,就得加入""
引号。
绑定对象属性
1 2 3
| <div v-bind="info"></div> -> <div age="12" name="Sam"></div>
|
1 2 3 4 5 6 7 8
| data(){ return { info: { age: 12, name: 'Sam' } } }
|
v-on
v-on内使用对象,可以传递多个对象属性进行与对应方法绑定,进行触发。
1
| <div v-on="{click: clickEvent}">点击</div>
|
1 2 3 4 5
| methods: { clickEvent() { console.log('click event handler...') } }
|
修饰符
v-on支持修饰符,修饰符相当于对事件进行了一些特殊的处理:
.stop - 调用 event.stopPropagation()。
.prevent - 调用 event.preventDefault()。
.capture - 添加事件侦听器时使用 capture 模式。
.self - 只当事件是从侦听器绑定的元素本身触发时才触发回调。
.{keyAlias} - 仅当事件是从特定键触发时才触发回调。
.once - 只触发一次回调。
.left - 只当点击鼠标左键时触发。
.right - 只当点击鼠标右键时触发。
.middle - 只当点击鼠标中键时触发。
.passive - { passive: true }模式添加侦听器。
v-if 与 v-show的区别
用法上
- v-show是不支持template
- v-show不可以和v-else一起使用
本质上
- v-show元素无论是否需要显示到浏览器上,它的DOM实际都是有渲染的,只是通过CSS的display属性来进行切换
- v-if当条件为false时,其对应元素压根不会被渲染到DOM上
开发中如何进行选择
- 如果我们开发的元素需要在显示和隐藏之间频繁的切换,那么使用v-show
- 如果不会频繁的发生切换,那么使用v-if
v-for
v-for遍历对象
1 2 3
| <p v-for="(value, key, index) in info"> {{value}}-{{key}}-{{index}} </p>
|
1 2 3 4 5
| info: { age: 12, name: 'Sam', Sex: 'Male' }
|
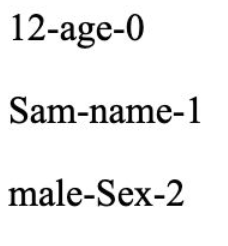
遍历数字
1
| <div v-for="num in 10">{{num}}</div>
|
计算属性 computed
computed:计算属性
字面意思:计算我们的属性,所以每次计算后,除非所依赖的属性发生了变化,不然他不会执行计算的,也就是它的缓存性。
与methods的区别
1 2 3 4 5 6
| <h2>{{fullName}}</h2> <h2>{{fullName}}</h2> <h2>{{fullName}}</h2> <h2>{{getFullName()}}</h2> <h2>{{getFullName()}}</h2> <h2>{{getFullName()}}</h2>
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| data() { return { firstName: 'Sam', lastName: 'Hbisedm' } }, computed: { fullName() { console.log('computed run...'); return `${this.firstName} ${this.lastName}` } }, methods: { getFullName() { console.log('methods run...'); return `${this.firstName} ${this.lastName}` } }
|
效果:
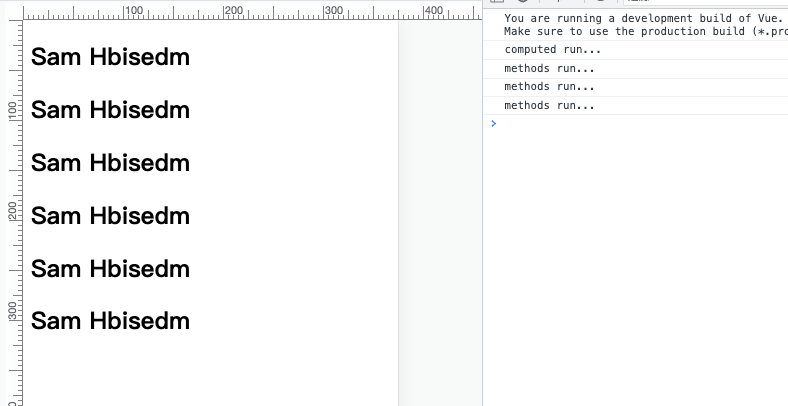
证明:
计算属性方法是具有缓存性的。
getter
1 2 3 4 5 6 7
| computed: {
fullName() { console.log('computed run...'); return `${this.firstName} ${this.lastName}` }, }
|
默认函数写法就是getter,使用对象写法可以写getter还可以写setter
1 2 3 4 5 6 7 8 9 10 11
| computed: { fullName: { get: function() { return `${this.firstName} ${this.lastName}` }, set: function(val) { this.firstName = val console.log(val) } } }
|
- 当程序调用
this.fullName = xxx
时,会自动调用fullName的setter方法
- 而setter方法会里面会改变
this.firstName
导致fullName的getter方法自动再次执行。
侦听器 watch
侦听某些数据的变化,需要使用watch。
1 2 3 4 5 6 7 8 9
| <template id="my-app"> <p>base data</p> <input v-model.lazy="message" /> <p>object</p> <p>{{ info.name }}</p> <button @click="changeObj">change obj</button> <button @click="changeAttr">change obj attr</button> </template>
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| const App = { template: '#my-app', data() { return { message: "Hello World", info: { name: 'Sam0', age: 23 } } }, watch: { message(newVal, oldVal) { console.log('new: ' + newVal, 'old: ' + oldVal); }, info(newVal, oldVal) { console.log('new: ' + newVal, 'old: ' + oldVal); }, }, methods: { changeObj() { this.info = { name: "Sam1" } }, changeAttr() { this.info.name = "Sam2" } } }
|
无论是基本数据还是引用类型的数据,只要改变了就会监听到。但如果是改变了对象里面的属性值,watch不会监听到,因为原来对象的内存地址没有进行修改。
那我们开发过程中需要监听对象里面的属性的情况下:
1 2 3 4 5 6 7 8 9
| info: { handler: function (newVal, oldVal) { console.log('new: ' + newVal, 'old: ' + oldVal); }, deep: true } "info.name": function(newVal, oldVal) { }
|
我们想watch里面某些正在监听的data在页面加载后立即执行一次(上面代码是当后面data改变后才执行watch)
1 2 3 4 5 6 7
| info: { handler: function (newVal, oldVal) { console.log('new: ' + newVal, 'old: ' + oldVal); }, deep: true, immediate: true }
|
一些其他用法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| watch: { message: '对应的方法名' } watch: { message: [ '对应方法名', { handler: function(newVal, oldVal) { } }, handler2(newVal, oldVal) { } ] }
|
- 可以传递字符串,使用对应的方法名
- 可以传递数组,使用对应的一系列回调
使用$watch
1 2 3 4 5 6 7 8 9 10
| created() { const unwatch = this.$watch("info", (newVal, oldVal) => { console.log('$watch newVal: ' + newVal, '$watch oldVal: ' + oldVal); }, { deep: true, immediate: true }) unwatch() }
|