#tag
Vue3-render函数的笔记
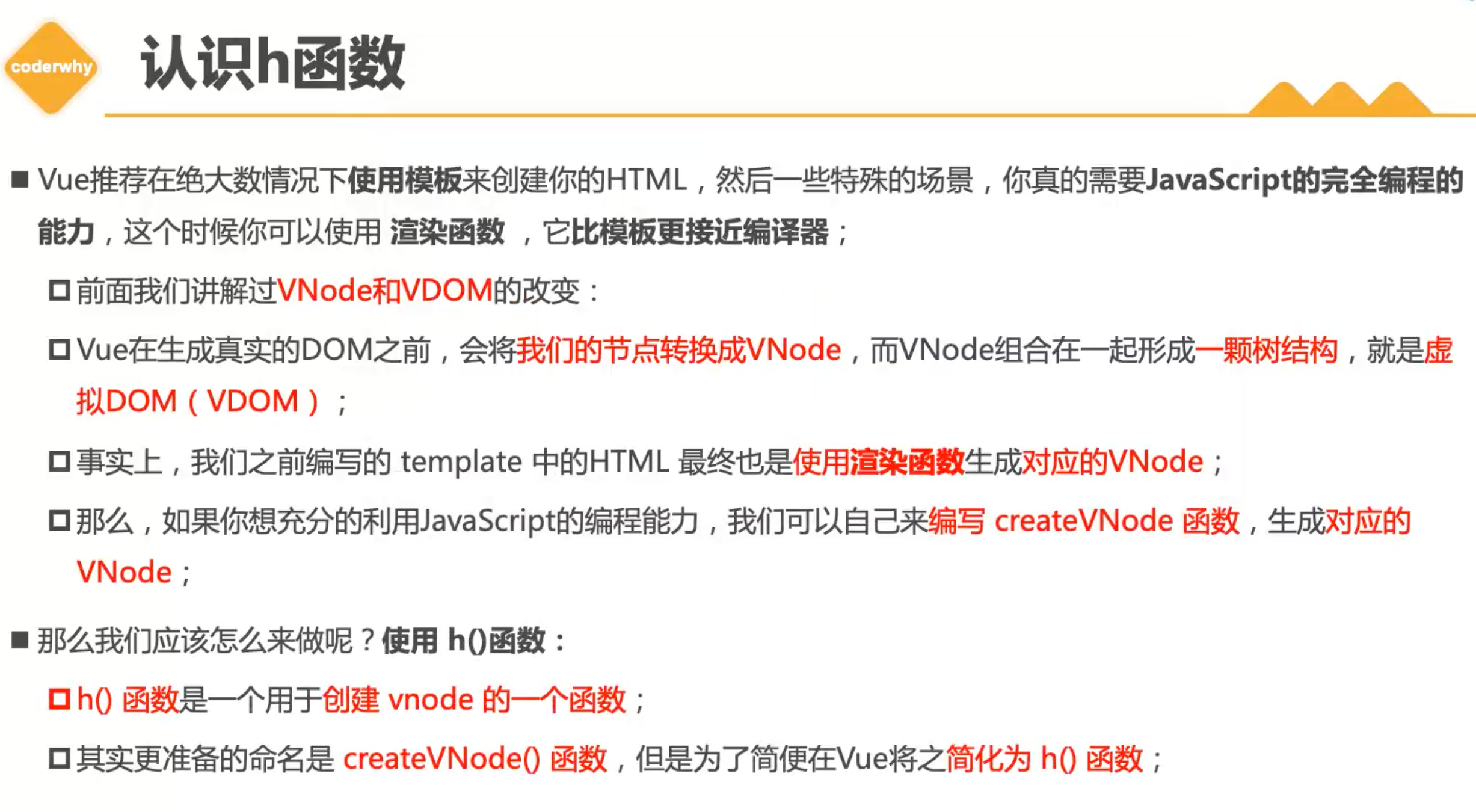
render的基本使用
| <script> import { h } from 'vue' export default { render() { return h('h2', {class: 'title'} ,'hello render') } } </script>
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| <script> import { h } from 'vue' export default { data() { return { counter: 0 } }, render() { return h('div', {class: 'title'}, [ h('h2', null, this.counter), h('button', { onClick: () => this.counter++ }, '+'), h('button', { onClick: () => this.counter-- }, '-') ]) } } </script>
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| import { ref, h } from 'vue' export default { setup() { const counter = ref(0) return { counter } }, render() { return h('div', {class: 'title'}, [ h('h2', null, this.counter), h('button', { onClick: () => this.counter++ }, '+'), h('button', { onClick: () => this.counter-- }, '-') ]) } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13
| import { ref, h } from 'vue' export default { setup() { const counter = ref(0) return () => { return h('div', {class: 'title'}, [ h('h2', null, counter.value), h('button', { onClick: () => counter.value++ }, '+'), h('button', { onClick: () => counter.value-- }, '-') ]) } }, }
|
props与slot
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| <script> import { h, ref } from 'vue' import Son from './04renderSlotS' export default { setup() { const count = ref('by Father') return () => { return h(Son, { msg: count }, { default: (props) => `default slot, get val: ${props}`, }) } } } </script>
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| <script> import { h} from 'vue' export default { props: { msg: { type: String, default: 'hello' } },
setup(props, {slots}) { console.log(props); return () => { return h('div', null, [ h('h2', null, `play render solt, get msg: ${props.msg.value}`), slots.default ? slots.default('from son') : '没有插槽' ]) } } } </script>
|
JSX
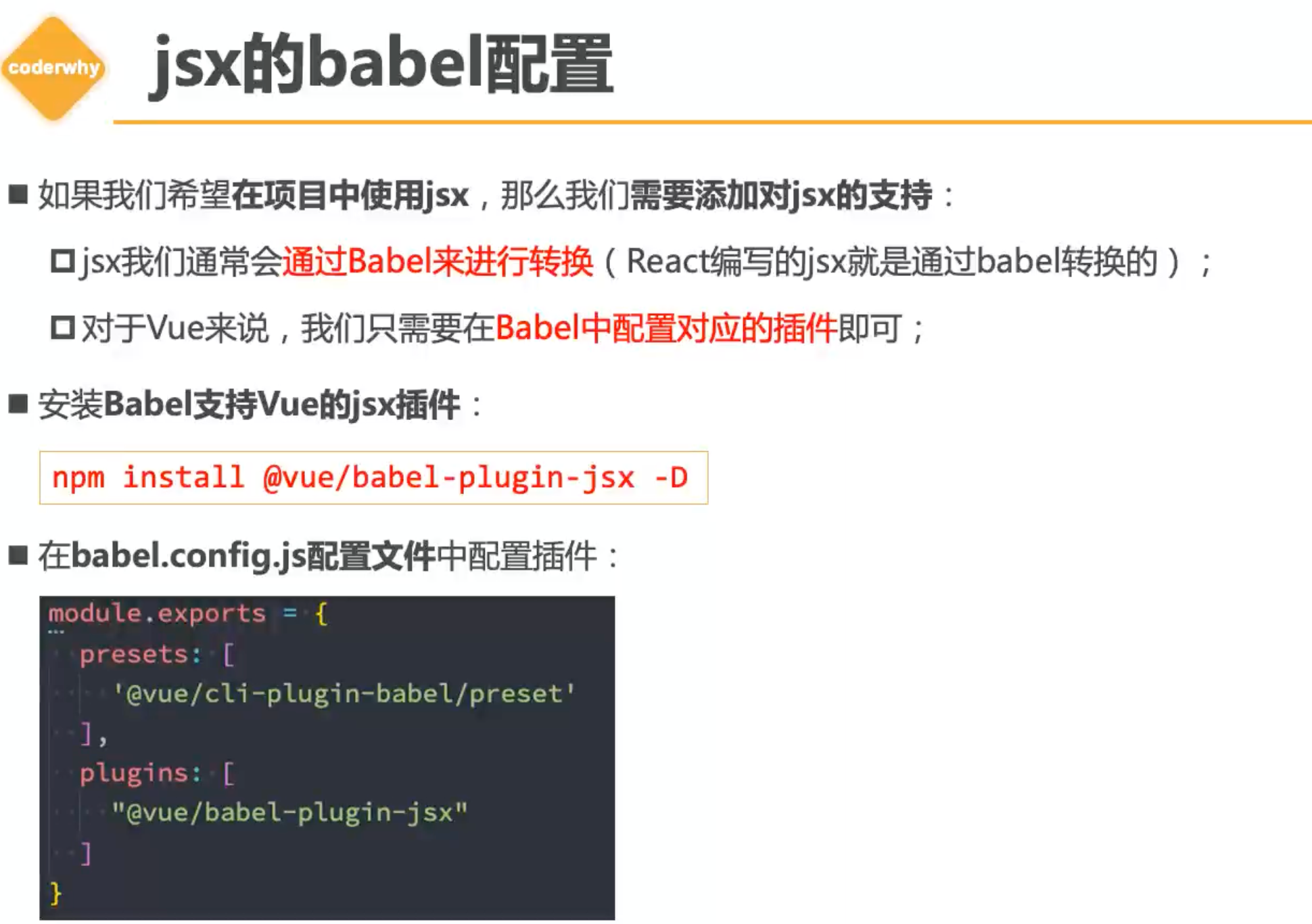
原理:jsx->babel->h()
因为h()的可读性太差了。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46
| <script> import JsxSon from './05jsxSon.vue' import { ref } from 'vue' export default { setup() { const className = 'title' const counter = ref(0) return { className, counter, } }, render() { const increment = () => { this.counter++ } const decrement = () => { this.counter-- } return ( <div> <h2 class={this.className}>hello jsx</h2> <p>counter: {this.counter}</p> <button onClick={increment}>+1</button> <button onClick={decrement}>-1</button> <JsxSon> { { default: props => { return <div>from jsx father</div> } } } </JsxSon> </div> ) } } </script>
<style scoped> .title { color: red; } </style>
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| <script> export default { render() { return ( <div class="son-area"> <p>jsx - son</p> <div>{this.$slots.default ? this.$slots.default() : '默认值...'}</div> </div> ) } } </script>
<style scoped> .son-area { background-color: slateblue; color: #fff; padding: 10px 0; margin: 10px 0; }
</style>
|
jsx优势:任意使用JavaScript代码、对比template灵活性更高、一般封装组件库才用。